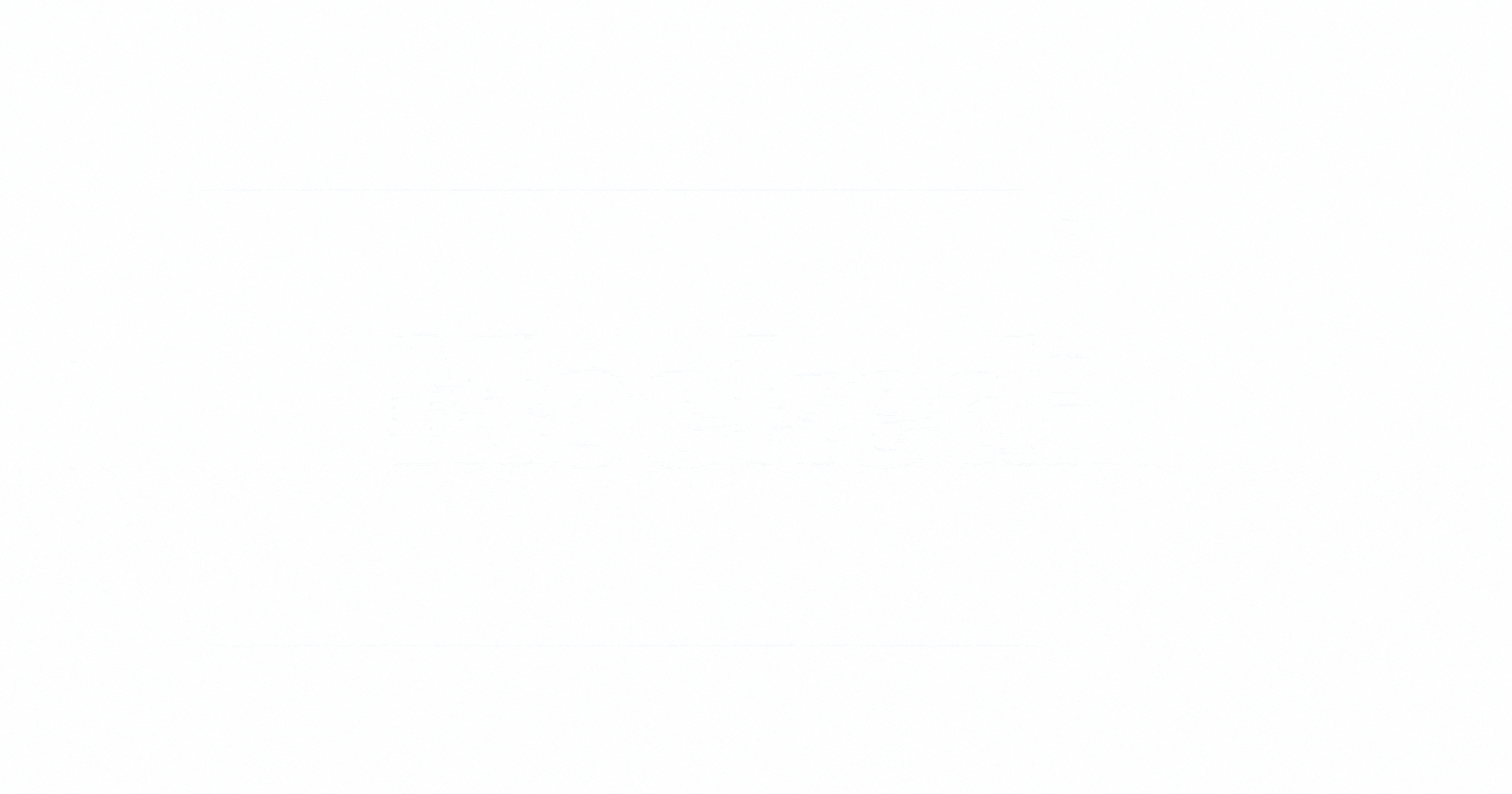
# Building Mockedin-Client: A Simplified LinkedIn Experience with React, Vite, JSON Server, and Concurrently
Table of Contents
-
๐ฌ Contact Me:
Github: https://github.com/willshepp28
Email: willsheppard.dev@gmail.com
LinkedIn: https://www.linkedin.com/in/will-sheppard-81a184145/Github Repo for this project:
https://github.com/willshepp28/Mockedin
Introduction
Welcome, developers! Introducing "Mockedin," a client-side project simulating key LinkedIn. This project is powered by React, Vite, JSON Server, and Concurrently, forming a robust environment for real-world development simulation.
Why Mockedin?
React: For interactive UI components.
Vite: For speedy development.
JSON Server: To mock REST APIs effortlessly.
Concurrently: To run frontend and backend simultaneously, saving development time.
Setting Up Mockedin-Client
With Yarn
Create new Vite-React project:
yarn create vite mockedin-client --template react
Go into your project folder:
cd mockedin-client
Install JSON Server and Concurrently:
yarn add json-server concurrently --dev
Optionally, install Axios:
yarn add axios
Update package.json scripts:
"scripts": { "dev": "concurrently \"vite\" \"yarn mock-api\"", "build": "vite build", "serve": "vite preview", "mock-api": "json-server --watch db.json --port 5000" }
Run your project:
yarn dev
With npm
Create new Vite-React project:
npm init vite@latest mockedin-client -- --template react
Navigate to your project folder:
cd mockedin-client
Install JSON Server and Concurrently:
npm install json-server concurrently --save-dev
Optional: Install Axios:
npm install axios
Update package.json scripts:
"scripts": { "dev": "concurrently \"vite\" \"npm run mock-api\"", "build": "vite build", "serve": "vite preview", "mock-api": "json-server --watch db.json --port 5000" }
Run your project:
npm run dev
Inside Mockedin-Client
Database File (db.json
)
Create a db.json
file to act as our faux LinkedIn database.
{
"linkedinUsers": [
{ "id": 1, "name": "John Doe", "position": "Developer" },
{ "id": 2, "name": "Jane Smith", "position": "Recruiter" },
{ "id": 3, "name": "Emily Johnson", "position": "CEO" },
{ "id": 4, "name": "Mike Brown", "position": "Sales" }
]
}
React Component (App.jsx
)
Here is how the React component looks to fetch and display data.
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const App = () => {
const [linkedinUsers, setLinkedinUsers] = useState([]);
useEffect(() => {
axios.get('http://localhost:5000/linkedinUsers').then(response => {
setLinkedinUsers(response.data);
});
}, []);
return (
<div>
{linkedinUsers.map(user => (
<div key={user.id}>{user.name} - {user.position}</div>
))}
</div>
);
};
In a nutshell:
db.json: A pretend LinkedIn database.
App.jsx: The orchestrator, getting LinkedIn profiles from our mock database and displaying them.
Concurrently: Our helper that ensures both the stage and the script are set before the show starts.
Conclusion
Mockedin-Client offers a rich development experience with React, Vite, JSON Server, and Concurrently. It is perfect for those wanting to develop LinkedIn-like features without worrying about real-world API limitations.
Project Folder Structure
Here's how the folder structure of Mockedin-Client looks:
mockedin-client/
โโโ node_modules/
โโโ public/
โ โโโ index.html
โโโ src/
โ โโโ App.jsx
โ โโโ main.jsx
โโโ db.json
โโโ package.json
โโโ README.md
Did you find this article helpful? Please consider following for more such content. Comments and questions are always welcome!